Have you ever wondered how smart homes automatically adjust temperature and humidity to keep you comfortable? Or how do weather stations collect real-time data for forecasts? A DHT11 sensor is one of the easiest ways to measure temperature and humidity, making it perfect for projects like climate monitoring, smart fans, greenhouse control, and weather stations.
The DHT11 sensor is a small, affordable device that gives accurate readings and works with almost any microcontroller, including Arduino. It uses a single wire to send data, making it easy to connect and use.
In this guide, I’ll walk you through everything you need to know about using the DHT11 sensor with an Arduino, including:
- How the sensor works
- How to wire it correctly
- Writing an easy Arduino code snippet
- Troubleshooting common problems
- Real-world applications
By the end of this tutorial, you’ll be able to integrate temperature and humidity monitoring into your projects. So, let’s learn!
What is the DHT11 Sensor?
The DHT11 is a digital sensor that measures:
- Temperature (°C) – Detects air temperature from 0°C to 50°C
- Humidity (%) – Measures moisture levels from 20% to 90%
Why Use the DHT11?
- Cheap & Easy to Use – Great for beginners
- Low Power Consumption – Works with batteries
- Decent Accuracy – Suitable for most DIY projects
It has four pins, but we only use three:
Pin | Function | Arduino Connection |
---|---|---|
VCC | Power Supply (3.3V/5V) | 5V |
Data | Sensor Output | Digital Pin 2 |
NC | Not Used | – |
GND | Ground | GND |
If you’re using a DHT11 module (with a small circuit board), it already has a pull-up resistor. If you’re using just the sensor, you’ll need to add a 10kΩ resistor between VCC and Data.
What You’ll Need
Here’s what you need for this project:
- Arduino Uno (or any other Arduino board)
- DHT11 Temperature and Humidity Sensor
- 10kΩ Resistor (if using the bare sensor)
- Jumper Wires
- Breadboard
How to Connect the DHT11 to Arduino
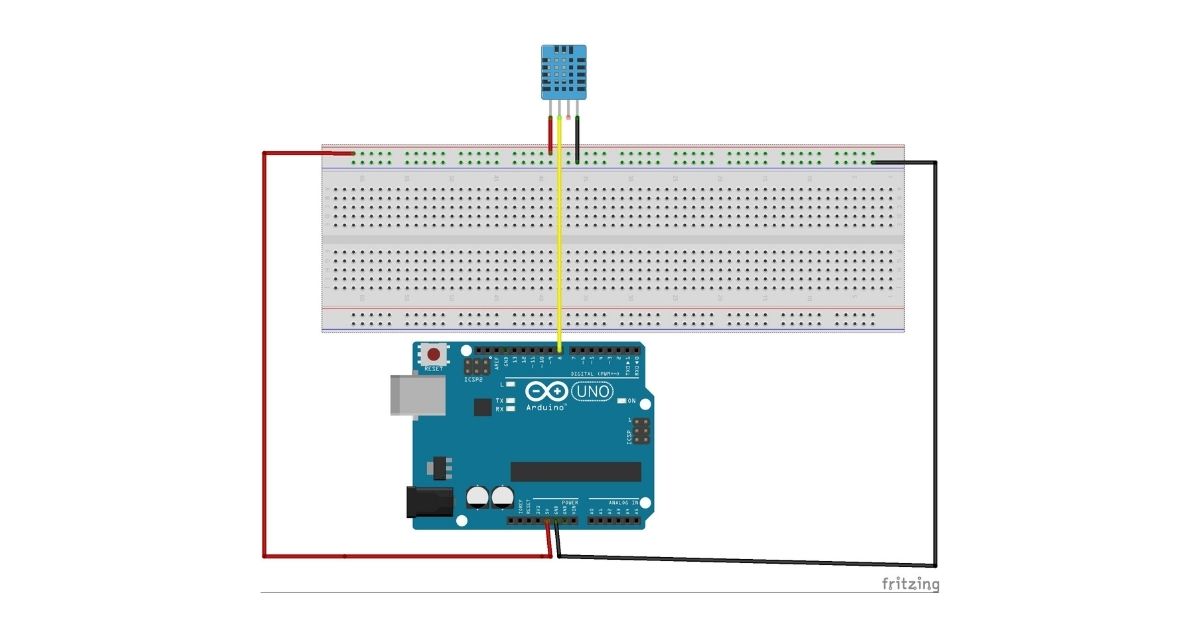
Here’s a simple wiring guide:
- DHT11 VCC → 5V on Arduino
- DHT11 GND → GND on Arduino
- DHT11 Data → Digital Pin 8 on Arduino
- 10kΩ Resistor → Between VCC and Data Pin (only if needed)
Once you’ve wired it up, we’re ready to write the code!
Installing the DHT Library
Before running the code, you need to install the DHT sensor library in Arduino IDE:
- Open Arduino IDE.
- Go to Sketch → Include Library → Manage Libraries.
- Search for the DHT sensor library by Adafruit.
- Click Install.
Now, let’s write the code!
Arduino Code to Read Temperature & Humidity
#include <DHT.h>
// Define the sensor pin and type
#define DHTPIN 8
#define DHTTYPE DHT11
// Create a DHT sensor object
DHT dht(DHTPIN, DHTTYPE);
void setup() {
Serial.begin(9600); // Start Serial Monitor
dht.begin(); // Initialize sensor
delay(2000); // Wait for the sensor to get stable readings
}
void loop() {
float humidity = dht.readHumidity();
float temperature = dht.readTemperature();
if (isnan(humidity) || isnan(temperature)) {
Serial.println("Failed to read from DHT sensor!");
return;
}
Serial.print("Humidity: ");
Serial.print(humidity);
Serial.print("% | Temperature: ");
Serial.print(temperature);
Serial.println("°C");
delay(2000); // Update every 2 seconds
}
How the Code Works
- Include the DHT Library → This makes it easy to read data from the sensor.
- Define the Sensor Pin & Type → The DHTPIN is set to 2, and the sensor type is DHT11.
- Initialize the Sensor →
dht.begin();
starts communication with the sensor. - Read Temperature & Humidity →
dht.readHumidity();
reads humidity (%)dht.readTemperature();
reads temperature (°C)
- Check for Errors → If the reading fails, it prints
"Failed to read from DHT sensor!"
. - Print Data to Serial Monitor → You can see real-time readings on your computer.
Testing the Setup
- Upload the code to your Arduino.
- Open the Serial Monitor (9600 baud rate).
- You should see readings like:
Humidity: 45.20% | Temperature: 27.50°C
Humidity: 46.00% | Temperature: 27.30°C
Common Issues & Fixes
- Getting “Failed to read from DHT sensor!”?
- Check your wiring.
- Make sure you selected DHT11 (not DHT22) in the code.
- Add a pull-up resistor (10kΩ) if needed.
- Readings seem wrong?
-
- Avoid placing the sensor near heat sources.
- Give it 2 seconds between readings to stabilize.
Where Can You Use This?
The DHT11 sensor is used in:
- Smart Homes – Automate AC, heaters, and fans.
- Weather Stations – Collect temperature & humidity data.
- Greenhouses – Keep plants in perfect condition.
- IoT Projects – Send data to the cloud for remote monitoring.
Conclusion
That’s it! You’ve successfully connected the DHT11 temperature and humidity sensor to an Arduino. Now you can measure climate conditions and use the data for home automation, weather monitoring, and more.
Want more tutorials? Visit Nirobit Learning Lab for more cool Arduino tutorials!