Are you working on an Arduino project that requires distance measurement? Whether you’re building an obstacle-avoidance robot, a smart parking assistant, or an automated liquid-level detector, the HC-SR04 Ultrasonic Sensor is one of the most effective and affordable sensors for the job.
This sensor is widely used in electronics projects because of its simplicity, accuracy, and low cost. It uses sound waves to detect objects and measure distances, making it ideal for robotics, automation, and security applications. Unlike infrared sensors, which can be affected by light and colour, the HC-SR04 works in almost any environment by using ultrasonic sound waves to measure distances accurately.
In this guide, we’ll cover everything you need to know to start using the HC-SR04 with an Arduino board. You’ll learn how the sensor works, how to wire it up, and how to write an Arduino program that reads and displays the measured distance.
By the end of this tutorial, you will have a fully functional Arduino setup capable of measuring distances and detecting obstacles efficiently.
How the HC-SR04 Ultrasonic Sensor Works
The HC-SR04 ultrasonic sensor measures distance by emitting ultrasonic waves and then detecting their reflection from an object. The sensor contains two main components:
- Trigger (Trig) Pin: Sends out an ultrasonic pulse.
- Echo Pin: Receives the reflected pulse.
- VCC: Connects to the 5V power supply.
- GND: Connects to ground.
When a signal is sent to the Trigger Pin, the sensor emits a short ultrasonic pulse (high-frequency sound wave). This pulse travels through the air until it hits an object. The Echo Pin then detects the reflected sound wave and measures the time it takes for the sound to return.
Using this time measurement, we calculate the distance of the object using the following formula:
Distance = (Speed of Sound * Time)/2
Since the speed of sound is 343 meters per second (m/s), we use this value in our calculations within the Arduino code.
Components Needed
To follow this tutorial, you will need:
- 1 x Arduino Board (Uno, Mega, or Nano)
- 1 x HC-SR04 Ultrasonic Sensor
- Jumper Wires
- Breadboard (optional)
- Computer with Arduino IDE installed
Wiring the HC-SR04 Sensor to Arduino
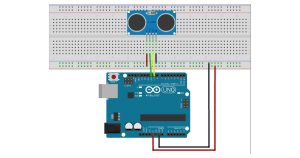
Now, let’s connect the HC-SR04 sensor to the Arduino Uno:
HC-SR04 Pin | Arduino Pin |
---|---|
VCC | 5V |
GND | GND |
Trig | D10 |
Echo | D9 |
If you are using an Arduino Mega or Nano, the wiring remains the same.
Arduino Code for Distance Measurement
Upload the following code to your Arduino to read distance values from the HC-SR04 sensor and display them in the Serial Monitor.
#define TRIG_PIN 10 // Connect to Trig pin of HC-SR04
#define ECHO_PIN 9 // Connect to Echo pin of HC-SR04
void setup() {
Serial.begin(9600); // Start serial communication
pinMode(TRIG_PIN, OUTPUT); // Set the trigger pin as output
pinMode(ECHO_PIN, INPUT); // Set the echo pin as input
}
void loop() {
long duration;
float distance;
// Clear the trigger pin before sending a pulse
digitalWrite(TRIG_PIN, LOW);
delayMicroseconds(2);
// Send a 10-microsecond pulse to trigger the sensor
digitalWrite(TRIG_PIN, HIGH);
delayMicroseconds(10);
digitalWrite(TRIG_PIN, LOW);
// Measure the pulse duration from the Echo pin
duration = pulseIn(ECHO_PIN, HIGH);
// Convert the time into distance in centimeters
distance = (duration * 0.0343) / 2;
// Print the distance to the Serial Monitor
Serial.print("Distance: ");
Serial.print(distance);
Serial.println(" cm");
delay(500); // Wait half a second before the next measurement
}
Code Walkthrough
- Defining Pins: The
#define
directive assigns names to the trigger and echo pins for easy reference. - Serial Communication Setup:
Serial.begin(9600);
starts serial communication at 9600 baud rate, allowing us to view sensor readings on the Serial Monitor. - Setting Pin Modes:
pinMode(TRIG_PIN, OUTPUT);
sets the trigger pin as an output, whilepinMode(ECHO_PIN, INPUT);
sets the echo pin as an input. - Sending the Ultrasonic Pulse:
- The trigger pin is set to LOW for 2 microseconds to clear any previous pulse.
- It is then set HIGH for 10 microseconds to send an ultrasonic pulse.
- It is then set to LOW again to stop the pulse.
- Measuring Echo Duration: The
pulseIn(ECHO_PIN, HIGH);
function waits for the pulse to return and measures the duration. - Calculating Distance: The formula
(duration * 0.0343) / 2
is used to convert time into distance in centimetres. - Displaying the Distance: The distance is printed to the Serial Monitor every 500 milliseconds.
Testing the Sensor
After uploading the code:
- Open the Serial Monitor in the Arduino IDE (set baud rate to 9600).
- Place an object in front of the sensor.
- You should see distance values updating in real time.
- Move the object closer or farther, and the values should change accordingly.
If you get inconsistent readings, ensure that:
- The sensor is properly wired.
- There are no obstacles interfering with the signal.
- The sensor is not too close to the object (less than 2 cm may not be detected).
Practical Applications
The HC-SR04 ultrasonic sensor is widely used in various real-world applications, including:
- Obstacle Avoidance Robots: Detects objects and avoid collisions.
- Parking Assist Systems: Helps cars detect nearby obstacles.
- Smart Waste Bins: Automatically open when a hand is detected.
- Liquid Level Monitoring: Measures the height of liquid in a container.
With minor modifications to the code, you can integrate this sensor into different Arduino projects for automation and security.
Conclusion
The HC-SR04 ultrasonic sensor is a simple yet powerful tool for distance measurement in Arduino projects. By following this guide, you have learned how to:
- Understand the working principle of the sensor.
- Connect it to an Arduino board.
- Write a simple program to measure distances.
- Apply it to real-world projects.
For more Arduino tutorials and high-quality electronic components, visit Nicrobit.